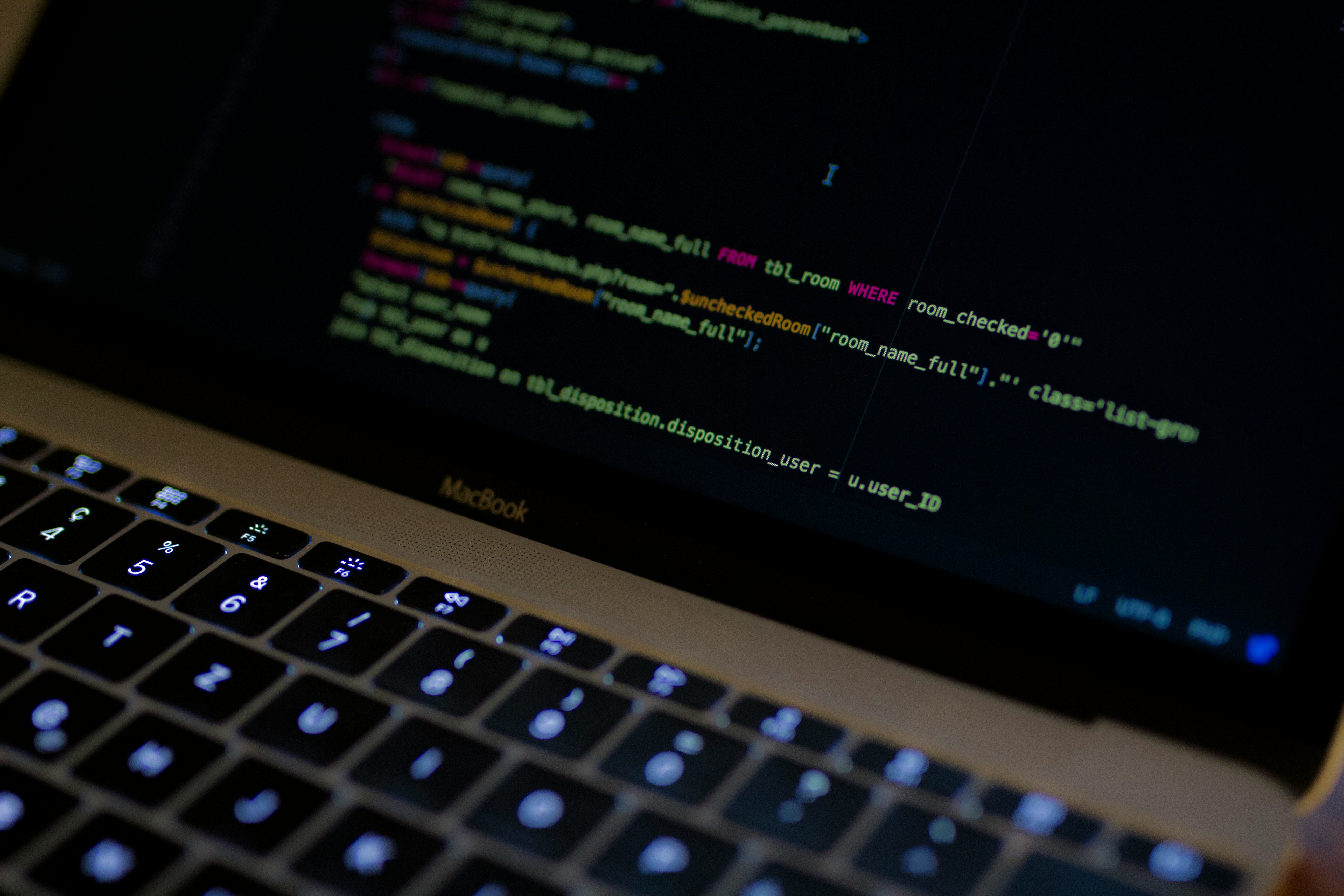
In this post, I want to discuss a tool in Yii2 that I believe is crucial for developers to understand and utilize effectively: database migration. This feature, inherent in Yii2, allows for the controlled, organized, and versioned management of database changes.
When starting a project from scratch, it is vital to employ this tool. Doing so means there's no need to maintain separate SQL files; instead, simply create the database and execute the migration command. Additionally, if migrating to a different database management system other than MySQL, changing the project's connection and running the migration command will automatically create the necessary tables in the new system. In essence, this tool makes projects more generic and portable, and the best tool if you are working with a team of developers.
There's a set of commands to handle database migrations. Let's start by analyzing the command to create a new table, such as the "category" table. The standard practice is to open the command window in your project and execute the following command:
php yii migrate/create create_category_table
Using the correct syntax guided by Yii2 documentation is crucial to save time. Yii2 will interpret the input and generate the table creation code, streamlining the process. Upon confirmation, the migration is ready, and if we go to the "console/migrations" directory in the Yii2 advanced project, it will reveal the generated file containing part of the code based on the correct syntax used.
The generated migration file will contain methods inherited from yii\db\Migration. These methods, documented in Yii2's official documentation, allow various database changes, from creating columns to adding foreign keys.
Maintaining safeUp (perform) and safeDown (undo) methods is advisable. For example, to add a "name" field to the "category" table, modify the safeUp method accordingly:
public function safeUp() {
$this->createTable(
'{{%category}}',
[ 'id' => $this->primaryKey(), 'name' => $this->string(255), ]
);
}
Adhering to the suggested method structure makes future modifications and reversions more manageable.
Once all desired changes are defined in the migration file, including data types for new fields, execute the migration command again:
php yii migrate
This command, previously used during the initial Yii2 project setup to generate the "user" table, will now apply the defined changes to the database.
Using php yii migrate/down, one can execute the down method, reverting changes made by previous migrations. Each migration is recorded in a dedicated database table ("migration"), facilitating versioning and tracking changes.
Yii2 documentation provides extensive resources in both English and Spanish for a comprehensive understanding and detailed usage.